Add UITextField to UIAlertController in Swift
In iOS UIAlertController is not only used to output messages to user but it can also accept input text from the user.
In this tutorial we will learn how to add UITextField to UIAlertController so lets start
1- Start by creating dragging a UIButton to your view controller then create the following function and connect it to the button
@IBAction func buttonHandler(_ sender: UIButton) { }
2- Inside the buttonHandler function create two instances of UITextField
var txtUsername: UITextField? var txtPassword: UITextField?
3- Create an instance of UIAlertController
let alert = UIAlertController(title: "Login", message: "Please enter your password and username", preferredStyle: .alert)
4- Now create an instance of UIAlertAction that reads the inputs from the two text fields and print them
let loginAction = UIAlertAction(title: "Login", style: .default) { (login) in if let username = txtUsername?.text { print("username is: \(username)") } else { print("No username") } if let password = txtPassword?.text { print("password is: \(password)") } else { print("No password") } }
5- Add two text fields to the alert object and configure them in the configuration block as follows
alert.addTextField { (usernameTextField) in txtUsername = usernameTextField txtUsername?.placeholder = "Enter your username" } alert.addTextField { (passwordTextField) in txtPassword = passwordTextField txtPassword?.isSecureTextEntry = true txtPassword?.placeholder = "Enter your password" }
6- add the action object created in step 4 to the alert object then present the alert
alert.addAction(loginAction) self.present(alert, animated: true, completion: nil)
7- Now run your app and tap the button, this should be the result
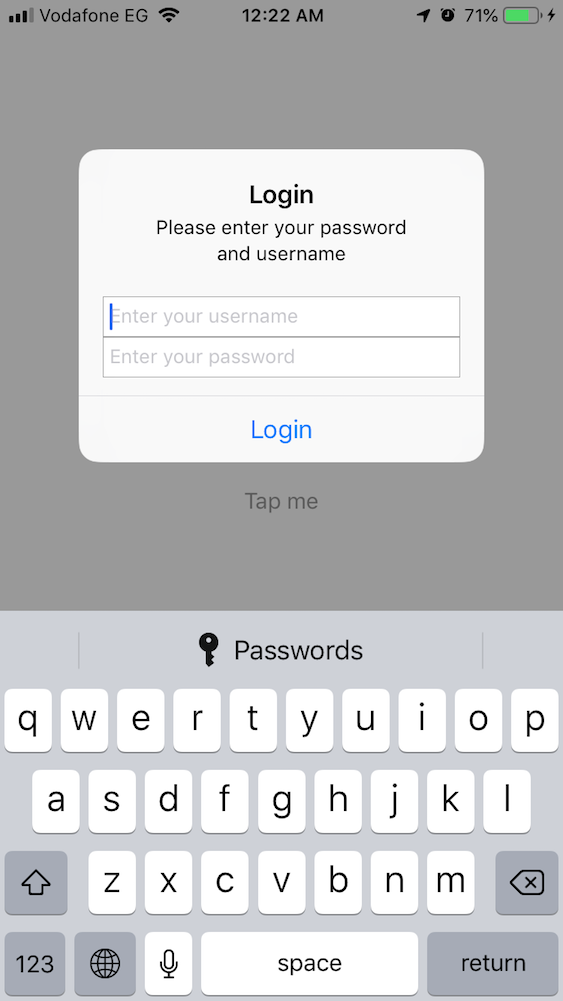
This is the complete code
@IBAction func buttonHandler(_ sender: UIButton) { var txtUsername: UITextField? var txtPassword: UITextField? let alert = UIAlertController(title: "Login", message: "Please enter your password and username", preferredStyle: .alert) let loginAction = UIAlertAction(title: "Login", style: .default) { (login) in if let username = txtUsername?.text { print("username is: \(username)") } else { print("No username") } if let password = txtPassword?.text { print("password is: \(password)") } else { print("No password") } } alert.addTextField { (usernameTextField) in txtUsername = usernameTextField txtUsername?.placeholder = "Enter your username" } alert.addTextField { (passwordTextField) in txtPassword = passwordTextField txtPassword?.isSecureTextEntry = true txtPassword?.placeholder = "Enter your password" } alert.addAction(loginAction) self.present(alert, animated: true, completion: nil) }